1-click AWS Deployment 1-click Azure Deployment
Overview
Ruby is an object-oriented programming language. Ruby is a dynamic programming language with a complex but at the same time communicative grammar. Ruby also has a core class library with a rich and powerful API.Ruby is stimulated by other low level and object-oriented programming languages like Lisp, Smalltalk, and Perl and uses syntax that is easy for C and Java programmers to learn.Ruby, a lively and open source programming language with a focus on simplicity and productivity, has a well-designed syntax that is natural to read and easy to write. Even though it’s easy to program in Ruby, it is not a simple language.Ruby is a pure object-oriented programming language. It was created in 1993 by Yukihiro Matsumoto of Japan.
Ruby is “A Programmer’s Best Friend”.
Ruby has features that are similar to those of Smalltalk, Perl, and Python. Perl, Python, and Smalltalk are scripting languages. Smalltalk is a true object-oriented language. Ruby, like Smalltalk, is a perfect object-oriented language. Using Ruby syntax is much easier than using Smalltalk syntax.
Ruby: Advanced Features
- Exceptions for handling errors.
- Garbage Collector.
- OS – independent threading, which allows you to write multi-threaded applications even on operating systems such as DOS.
- You can write extensions to Ruby in C.
Why you should go for Ruby?
- Ruby is a server-side scripting language.
- Ruby can be embedded into HTML.
- Ruby has similar syntax to that of many programming languages like C and Java.
- Ruby supports mostly all the platforms like Windows, Mac and Linux.
- Ruby can be easily connected to Oracle, MySQL, DB2.
Using the Ruby Interactive Shell
It is also called irb. It allows us to type ruby expressions or statements. Open the command prompt and type irb. (both Windows and Macintosh).
As you can see in the above screen, we can write ruby expressions and statements. The texts entered within quotes are called String. We can perform Concatenation Operation (concatenation – Joining two Strings) on two strings.
We can store the value to the variable simply like,
name = “Welcome to Ruby!”
Ruby is a case sensitive programming language. So, name is different from Name and NAME.
We can also perform some complicated operations. See the below code snippet which prints numbers from 1 to 9.
i = 1 while i < 10 print i end
Output :
Whoa!! What just happened? You might be confused after seeing the output. We said it prints 1 to 9. But it’s showing all 1’s and that also too many. This is because the variable i
was not incremented. Press Ctrl + C to terminate the execution.
The following code prints from 1 to 9
i = 1 while i < 10 print i i += 1 end
We can also define functions in irb
. A function is a set of statement(s) to perform a particular task. Here is a function that returns square of a number.
def square(n) return n * n end
Calling the function: square (4)
Calling this function and passing 4 as argument returns 16 by performing the operation 4 * 4
. Likewise, a function to find the area of a circle.
def circle(radius) return radius * radius * 3.14 end
Calling the function: circle (5)
and passing 5 as argument. This function returns 78.5 by multiplying 5 * 5 * 3.14
Ruby Scripts
We are going to run ruby from a text editor rather that a Command Prompt. You can use any text editor like Notepad, Notepad++, Sublime, Brackets. Ruby scripts have .rb extension. So, you have to save the file with the extension .rb
. Now, in your editor enter the following code and save it with any name with .rb
extension:
puts "Welcome to Ruby!"
Let’s save this as first.rb. To run the program, go to Command Prompt, and type ruby first.rb
. You can also run by simply typing first.rb
as well, if during installation, “Add Ruby executables to your PATH” option was checked.
Likewise, you can use any text editor in Macintosh and follow the same procedures as in Windows.
Comments in Ruby
Comments
are non-executable statements in Ruby
. Comments
are hidden from Ruby Interpreter so that these lines are ignored. They are used for increasing readability of the program. We can write comments anywhere in a program except inside a variable, because Ruby Interpreter considers it as a String.
Ruby: Single line Comment
Single line comment
is created using #(Hash)
sign in Ruby.
print “Hello World” #Used to Display the Text
Whenever, Ruby
finds # on a line, everything after that will be ignored.
Ruby: Multiple line Comment
Multiple line comments are created using the code blocks =begin / =end
=begin This is used to illustrate multiple line comments in Ruby language. =end
The statements inside this block are ignored by the Ruby Interpreter.
Valid example of Commenting:
Puts “4 * 6 = #{4*6}” #Multiplies 4 with 6 and Displays result
Display Data using print()
and puts()
Now, we will learn how to display data to the screen. For this purpose, Ruby provides two functions :
puts()
print()
Ruby: puts()
method
puts
adds a new line automatically at the end of the data.
puts ("hello, world") puts "hello, world"
You can use both the ways to display the data. To print multiple strings in a single puts statement use the below approach. It will add a newline character at the end of each string.
puts "hello, world", "Goodbye, world"
Also try the below example :
puts "practice", "makes", "a", "man", "perfect"
Ruby: print()
method
print
doesn’t add a new line at the end.
print "hello, world"
If you want to include new line at the end of the data using print method, then you have to include special character \n
for new line.
print "hello, world\n" print "practice\n", "makes\n", "a\n", "man\n", "perfect\n"
You can also display the numeric data using these functions.
puts(1) print(1)
Also you can evaluate the expression and display the result.
puts (2+2) print(2+2)
Getting Data from User using gets
in Ruby
To make the program to interact with user, a program usually needs input from the users. To do this, we can use gets
method. gets
function takes input from the keyboard in string format and stores the value in the variables.
name = gets
This statement takes a string input from the user and stores it in the variable called name.
It also appends a \n
new line character at the end of the input entered by the user.
So, when you type the variable name, it displays what is stored in it. When you display using print
function, it displays the text entered by the user along with a new line. To remove the newline, you can use a function called chomp
. The following statement removes the newline from the input entered by the user.
print name.chomp
The following code performs addition of two whole numbers with the numbers provided by the user:
number1 = gets number2 = gets (Integer)number1 + Integer(number2)
Integer()
part of the code looks tricky for you now.
As mentioned before, gets
method gets input in string format. So, conversion to whole number is required before arithmatic operations could be perfomed on the data. This can be done using Integer()
function.
If you don’t convert those variables into integer, it will concatenate the two string instead of performing arithmatic addition,. Do you remember concatenating two strings which we have seen earlier in Chapter 1? Likewise, to add two decimal numbers, look at the following code:
num1 = gets num2 = gets float(num1) + float(num2)
Operators and Expressions in Ruby
We are going to look at the different operators
that ruby contains and how to use them in the expressions
.
Ruby: Addition Operator
+
symbol is used. It is both binary and unary operator. As the name suggests a Binary operator
needs two operands/values on the either side of the operator to perform an operation and a unary operator needs just a single operand. Unary Plus serves no purpose, it is present just for the symmetry with unary minus.
For example : 1 + 2 //binary addition value = +3 //unary plus
Ruby: Subtraction Operator
-
symbol is used. You can use unary minus to reverse sign of a variable.
For Example : 23.2 - 2.2 //binary subtraction value = -3 //unary minus
Ruby: Multiplication Operator
*
symbol is used. Perfoms Multiplication on two numeric operands.
For Example : 122 * 3.14
Ruby: Division Operator
/
symbol is used. Returns result of devision of the first numeric operand by second operand.
For Example : 18 / 3
Ruby: Modulo Operator
Returns remainder after division.
For Example : 13 % 2 //returns 1
Ruby: Exponential Operator
If you want to raise x
to the power of y
(i.e) x ^ y
.
It is done by x ** y
2 raised to the power 4 returns 16.
Ruby: Operator Precedence
Operators
have some order of precedence
which determines the order in which an expression will be evaluated.
Consider this example, 1 + 2 * 3
We might think that the 1 + 2 is performed and the result 3 will be multiplied by 3 and gives 9. But the multiplication, division and exponential operator have higher precedence than addition and subtraction operators. Therefore, 2 *3 is performed first and the result is added to 1 and gives 7 as an answer.
However, we can modify the order of precedence by putting a subexpression in parentheses. In the expression 1 + 2 * 3, if 1 + 2 need to be performed first, put that expression in parentheses.
(1 + 2) * 3 this expression produces 9
Ruby: Relational Operators
Relational operators
are used for comparisons. They return Boolean values
. We compare two values whether they are equal, not equal, less than, greater than, less than or equal to and greater than or equal to.
==
sign is used. Used to check whether two numbers are equal or not.
1 == 1 returns true because 1 is equal to 1.
<
– Less than
>
– Greater than
<=
– Less than or equal to
>=
– Greater than or equal to
Less than operator checks whether a number is less than the another number, if yes it returns true else returns false. Less than or equal to operator checks whether a number is less than to another number and also checks whether a number is equal to another number, if any one of the condition is correct it returns true else returns false. Greater than and Greater than or equal to does the same and checks whether it is greater.
Another way to compare two values is using General comparison operator. It returns 0(zero), -1 or +1 depending on the operands. If both the values are equal it returns zero, if the first operand is less than the second operand it returns -1 and +1 if the first operand is greater than the second.
The General Comparison operator is <=>(< = >).
Relational operators
can be used with strings also.
If it is same it returns true. You could see that it returns false for the operation ‘Apple’ == ‘apple’ This is because Ruby is case-sensitive and one of the word has Uppercase A
while the other word has lowercase a
. We can also use General comparison operator with strings.
When comparing ‘car’ and ‘car’ it returned 0 since both are equal. When comparing ‘cab’ with ‘car’ it returned -1 because the 3rd letter of the word ‘b’ in ‘cab’ is less than ‘r’ in ‘car’.
Ruby: Logical Operators
Logical operators
allow you to combine two or more relational expressions and returns Boolean
value.
- AND operator:
It returns true when all of the expressions are true and returns false if even one of the expression evaluates to false. You could use this operator using and
or &&
.
It returned true for the expression salary == 10 && hours == 40 because we’ve initialized the value of the variables salary and hours as 10 and 40. It will return false for all other cases.
- OR operator:
OR
operator returns true when any one condition/expression is true and returns false only when all of them are false. You can use this operator using or
(or) ||
You can see that even when only one expression is true OR operator returned true.
- NOT operator:
NOT
operator negates a relational expression. You can use not
(or) !
for this operator.
In the first expression it returned false because the expression salary == 10 returns true and the not operator negates true and returns false. Likewise, the expression salary < 10 returns false and not operator negates and returns true.
Ruby provides a feature called string interpolation that lets you substitute the result of Ruby code into the middle of a string.
- Interpolation works within double-quoted Ruby strings.
- Anywhere you want within the string, you can include an interpolation marker. A marker consists of a hash mark (also known as a pound sign), an opening curly brace, and a closing curly brace:
#{}
- You can include any Ruby code you want between the curly braces.
- The code will be evaluated, the result will be converted to a string, and the resulting string will be substituted for the interpolation marker within the containing string.
- Lets us concatenate values like numbers with strings.
- Lets us embed simple math operations.
- Lets us embed the values of variables.
- We can include multiple interpolation markers into a single string.
- No interpolation in single-quoted strings.
$ irb
2.3.0 :001 > "aaa #{Time.now} bbb"
=> "aaa 2017-09-02 19:12:52 -0700 bbb"
2.3.0 :002 > "a string #{1}"
=> "a string 1"
2.3.0 :003 > "The answer is #{1 + 2}"
=> "The answer is 3"
2.3.0 :004 > name = "Jay"
=> "Jay"
2.3.0 :005 > "Hello, #{name}!"
=> "Hello, Jay!"
2.3.0 :006 > "#{Time.now} #{name}"
=> "2017-09-02 19:15:08 -0700 Jay"
2.3.0 :007 > 'Hello, #{name}!'
=> "Hello, \#{name}!"
Widget store code
- Let’s try improving our widget store code using string interpolation.
- We’ll remove the plus signs to combine all the concatenated strings into one.
- In their place, we’ll add an interpolation marker that inserts the value of the
answer
variable:puts "You entered #{answer} widgets"
- It’s easy to remember to put spaces surrounding the interpolation marker because it would look funny if we didn’t.
def ask(question)
print question + " "
gets
end
puts "Welcome to the widget store!"
answer = ask("How many widgets are you ordering?")
puts "You entered #{answer} widgets"
You can see in the output that it’s inserting the value of the answer
variable into the string, and it’s surrounded by spaces as it should be.
You entered 10
widgets
Ruby Methods
Methods are also called as Functions. Methods are used to execute specific set of instructions
For example,
- File Menu in Word has different operations like Save, Open etc.,
- Save is used to specifically save the document and contains corresponding code for it.
- Open command is used to open the document.
Functions take arguments and have return type.
Arguments are like an input given to the function and return type is the output of the function.
Return type can be two types in ruby:
- By using the expression
- By using
return
keyword.
Syntax of method:
def methodname(arguments) statements return end
In the above code, there is a method named square
with one argument. A variable named number
is assigned with the value of 3. Another variable called numbersqr
is assigned with the value returned by the function. Here, we call the function by specifying the name and passing the argument.
square(number). It is called function calling statement.
The variable number
is passed to the function which is assigned to the local variable num
in the function, the number is multiplied and the function returns the value which is assigned to the variable numbersqr
.
This is the output of the above program :
The value 9 is returned from the function and assigned to variable numbersqr
and displayed using print
method. In this example, the return type of the function is using expression.
Ruby Method with more than one parameter
In this program, there are two methods square
and power
. Power method takes two arguments. This method returns the value after raising the value present in the base variable to the value present in the exp
variable. This method explicitly returns the value. That is, the value is returned using return
keyword.
def power (base, exp) product = 1 while exp > 0 product *= base exp -= 1 end return product end
When this function is called by passing the following parameters: power (3, 3)
. It returns the value of 3 raised to the power 3.
In this program, the function calling statement is written inside print method. This specifies that function called be anywhere in the program.
First, the square method is called, it returns the value and stored in the variable numbersqr
. Now, when calling power method, we are passing the variables numbersqr
and number
. Therefore, the value of numbersqr ^ number
is returned.
In our case, 9^3
or 9*9*9
which is 729 is returned from the function.
–Ruby is a dynamic, open source programming language with a focus on simplicity and productivity.Ruby is a dynamic, reflective, object-oriented, general-purpose programming language. It has an elegant syntax that is natural to read and easy to write.It supports multiple programming paradigms, including functional, object-oriented, and imperative. It also has a dynamic type system and automatic memory management.
Ruby Class and Object
Here, we will learn about Ruby objects and classes. In object-oriented programming language, we design programs using objects and classes.
Object is a physical as well as logical entity whereas class is a logical entity only.
Ruby Object
Object is the default root of all Ruby objects. Ruby objects inherit from BasicObject (it is the parent class of all classes in Ruby) which allows creating alternate object hierarchies.Object mixes in the Kernel module which makes the built-in Kernel functions globally accessible.
Creating object
Objects in Ruby are created by calling new method of the class. It is a unique type of method and predefined in the Ruby library.Ruby objects are instances of the class.
Syntax:
- objectName = className.new
Example:
We have a class named Java. Now, let’s create an object java and use it with following command,
- java = Java.new(“John”)
Ruby Class
Each Ruby class is an instance of class Class. Classes in Ruby are first-class objects.
Ruby class always starts with the keyword class followed by the class name. Conventionally, for class name we use CamelCase. The class name should always start with a capital letter. Defining class is finished with end keyword.
Syntax:
- class ClassName
- codes…
- end
Example:
In the above example, we have created a class Home using class keyword. The @love is an instance variable, and is available to all methods of class Home.
Ruby Variables
Ruby variables are locations which hold data to be used in the programs. Each variable has a different name. These variable names are based on some naming conventions. Unlike other programming languages, there is no need to declare a variable in Ruby. A prefix is needed to indicate it.
There are four types of variables in Ruby:
⦁ Local variables
⦁ Class variables
⦁ Instance variables
⦁ Global variables
Ruby Data types
Data types represents a type of data such as text, string, numbers, etc. There are different data types in Ruby:
⦁ Numbers
⦁ Strings
⦁ Symbols
⦁ Hashes
⦁ Arrays
⦁ Booleans
Ruby If-else Statement
The Ruby if else statement is used to test condition. There are various types of if statement in Ruby.
⦁ if statement
⦁ if-else statement
⦁ if-else-if (elsif) statement
⦁ ternay (shortened if statement) statement
Ruby if statement
Ruby if statement tests the condition. The if block statement is executed if condition is true.
⦁ if (condition)
⦁ //code to be executed
⦁ end
Ruby if else
Ruby if else statement tests the condition. The if block statement is executed if condition is true otherwise else block statement is executed.
Syntax:
⦁ if(condition)
⦁ //code if condition is true
⦁ else
⦁ //code if condition is false
⦁ end
Ruby if else if (elsif)
Ruby if else if statement tests the condition. The if block statement is executed if condition is true otherwise else block statement is executed.
Syntax:
⦁ if(condition1)
⦁ //code to be executed if condition1is true
⦁ elsif (condition2)
⦁ //code to be executed if condition2 is true
⦁ else (condition3)
⦁ //code to be executed if condition3 is true
⦁ end
Ruby Case Statement
In Ruby, we use ‘case’ instead of ‘switch’ and ‘when’ instead of ‘case’. The case statement matches one statement with multiple conditions just like a switch statement in other languages.
Syntax:
⦁ case expression
⦁ [when expression [, expression …] [then]
⦁ code ]…
⦁ [else
⦁ code ]
⦁ end
Ruby/DBI
DBI stands for Database Independent Interface for Ruby, which means DBI provides an abstraction layer between the Ruby code and the underlying database, allowing you to switch database implementations really easily. It defines a set of methods, variables, and conventions that provide a consistent database interface, independent of the actual database being used.
DBI can interface with the following −
- ADO (ActiveX Data Objects)
- DB2
- Frontbase
- mSQL
- MySQL
- ODBC
- Oracle
- OCI8 (Oracle)
- PostgreSQL
- Proxy/Server
- SQLite
- SQLRelay
Architecture of a DBI Application
DBI is independent of any database available in the backend. You can use DBI whether you are working with Oracle, MySQL or Informix, etc. This is clear from the following architecture diagram.
The general architecture for Ruby DBI uses two layers −
- The database interface (DBI) layer. This layer is database independent and provides a set of common access methods that are used the same way regardless of the type of database server with which you’re communicating.
- The database driver (DBD) layer. This layer is database dependent; different drivers provide access to different database engines. There is one driver for MySQL, another for PostgreSQL, another for InterBase, another for Oracle, and so forth. Each driver interprets requests from the DBI layer and maps them onto requests appropriate for a given type of database server.
Prerequisites
If you want to write Ruby scripts to access MySQL databases, you’ll need to have the Ruby MySQL module installed.
Obtaining and Installing Ruby/DBI
Before starting this installation make sure you have the root privilege. Now, follow the steps given below −
Step 1
$ tar zxf dbi-0.2.0.tar.gz
Step 2
Go in distribution directory dbi-0.2.0 nd configure it using the setup.rb script in that directory. The most general configuration command looks like this, with no arguments following the config argument. This command configures the distribution to install all drivers by default.
$ ruby setup.rb config
To be more specific, provide a –with option that lists the particular parts of the distribution you want to use. For example, to configure only the main DBI module and the MySQL DBD-level driver, issue the following command −
$ ruby setup.rb config --with = dbi,dbd_mysql
Step 3
Final step is to build the driver and install it using the following commands −
$ ruby setup.rb setup $ ruby setup.rb install
Database Connection
Assuming we are going to work with MySQL database, before connecting to a database make sure of the following −
- You have created a database TESTDB.
- You have created EMPLOYEE in TESTDB.
- This table is having fields FIRST_NAME, LAST_NAME, AGE, SEX, and INCOME.
- User ID “testuser” and password “test123” are set to access TESTDB.
- Ruby Module DBI is installed properly on your machine.
- You have gone through MySQL tutorial to understand MySQL Basics.
Following is the example of connecting with MySQL database “TESTDB”
#!/usr/bin/ruby -w require "dbi" begin # connect to the MySQL server dbh = DBI.connect("DBI:Mysql:TESTDB:localhost", "testuser", "test123") # get server version string and display it row = dbh.select_one("SELECT VERSION()") puts "Server version: " + row[0] rescue DBI::DatabaseError => e puts "An error occurred" puts "Error code: #{e.err}" puts "Error message: #{e.errstr}" ensure # disconnect from server dbh.disconnect if dbh end
While running this script, it produces the following result at our Linux machine.
Server version: 5.0.45
If a connection is established with the data source, then a Database Handle is returned and saved into dbh for further use otherwise dbh is set to nil value and e.err and e::errstr return error code and an error string respectively.
Finally, before coming out it, ensure that database connection is closed and resources are released.
INSERT Operation
INSERT operation is required when you want to create your records into a database table.
Once a database connection is established, we are ready to create tables or records into the database tables using do method or prepare and execute method.
Using do Statement
Statements that do not return rows can be issued by invoking the do database handle method. This method takes a statement string argument and returns a count of the number of rows affected by the statement.
dbh.do("DROP TABLE IF EXISTS EMPLOYEE") dbh.do("CREATE TABLE EMPLOYEE ( FIRST_NAME CHAR(20) NOT NULL, LAST_NAME CHAR(20), AGE INT, SEX CHAR(1), INCOME FLOAT )" );
Similarly, you can execute the SQL INSERT statement to create a record into the EMPLOYEE table.
#!/usr/bin/ruby -w require "dbi" begin # connect to the MySQL server dbh = DBI.connect("DBI:Mysql:TESTDB:localhost", "testuser", "test123") dbh.do( "INSERT INTO EMPLOYEE(FIRST_NAME, LAST_NAME, AGE, SEX, INCOME) VALUES ('Mac', 'Mohan', 20, 'M', 2000)" ) puts "Record has been created" dbh.commit rescue DBI::DatabaseError => e puts "An error occurred" puts "Error code: #{e.err}" puts "Error message: #{e.errstr}" dbh.rollback ensure # disconnect from server dbh.disconnect if dbh end
Using prepare and execute
You can use prepare and execute methods of DBI class to execute the SQL statement through Ruby code.
Record creation takes the following steps −
- Preparing SQL statement with INSERT statement. This will be done using the prepare method.
- Executing SQL query to select all the results from the database. This will be done using the execute method.
- Releasing Statement handle. This will be done using finish API
- If everything goes fine, then commit this operation otherwise you can rollback the complete transaction.
Following is the syntax to use these two methods −
sth = dbh.prepare(statement) sth.execute ... zero or more SQL operations ... sth.finish
These two methods can be used to pass bind values to SQL statements. There may be a case when values to be entered is not given in advance. In such a case, binding values are used. A question mark (?) is used in place of actual values and then actual values are passed through execute() API.
Following is the example to create two records in the EMPLOYEE table −
#!/usr/bin/ruby -w require "dbi" begin # connect to the MySQL server dbh = DBI.connect("DBI:Mysql:TESTDB:localhost", "testuser", "test123") sth = dbh.prepare( "INSERT INTO EMPLOYEE(FIRST_NAME, LAST_NAME, AGE, SEX, INCOME) VALUES (?, ?, ?, ?, ?)" ) sth.execute('John', 'Poul', 25, 'M', 2300) sth.execute('Zara', 'Ali', 17, 'F', 1000) sth.finish dbh.commit puts "Record has been created" rescue DBI::DatabaseError => e puts "An error occurred" puts "Error code: #{e.err}" puts "Error message: #{e.errstr}" dbh.rollback ensure # disconnect from server dbh.disconnect if dbh end
If there are multiple INSERTs at a time, then preparing a statement first and then executing it multiple times within a loop is more efficient than invoking do each time through the loop.
READ Operation
READ Operation on any database means to fetch some useful information from the database.
Once our database connection is established, we are ready to make a query into this database. We can use either do method or prepare and execute methods to fetch values from a database table.
Record fetching takes following steps −
- Preparing SQL query based on required conditions. This will be done using the prepare method.
- Executing SQL query to select all the results from the database. This will be done using the execute method.
- Fetching all the results one by one and printing those results. This will be done using the fetch method.
- Releasing Statement handle. This will be done using the finish method.
Following is the procedure to query all the records from EMPLOYEE table having salary more than 1000.
#!/usr/bin/ruby -w require "dbi" begin # connect to the MySQL server dbh = DBI.connect("DBI:Mysql:TESTDB:localhost", "testuser", "test123") sth = dbh.prepare("SELECT * FROM EMPLOYEE WHERE INCOME > ?") sth.execute(1000) sth.fetch do |row| printf "First Name: %s, Last Name : %s\n", row[0], row[1] printf "Age: %d, Sex : %s\n", row[2], row[3] printf "Salary :%d \n\n", row[4] end sth.finish rescue DBI::DatabaseError => e puts "An error occurred" puts "Error code: #{e.err}" puts "Error message: #{e.errstr}" ensure # disconnect from server dbh.disconnect if dbh end
This will produce the following result −
First Name: Mac, Last Name : Mohan Age: 20, Sex : M Salary :2000 First Name: John, Last Name : Poul Age: 25, Sex : M Salary :2300
There are more short cut methods to fetch records from the database. If you are interested then go through the Fetching the Result otherwise proceed to the next section.
Update Operation
UPDATE Operation on any database means to update one or more records, which are already available in the database. Following is the procedure to update all the records having SEX as ‘M’. Here, we will increase AGE of all the males by one year. This will take three steps −
- Preparing SQL query based on required conditions. This will be done using the prepare method.
- Executing SQL query to select all the results from the database. This will be done using the execute method.
- Releasing Statement handle. This will be done using the finish method.
- If everything goes fine then commit this operation otherwise you can rollback the complete transaction.
#!/usr/bin/ruby -w require "dbi" begin # connect to the MySQL server dbh = DBI.connect("DBI:Mysql:TESTDB:localhost", "testuser", "test123") sth = dbh.prepare("UPDATE EMPLOYEE SET AGE = AGE + 1 WHERE SEX = ?") sth.execute('M') sth.finish dbh.commit rescue DBI::DatabaseError => e puts "An error occurred" puts "Error code: #{e.err}" puts "Error message: #{e.errstr}" dbh.rollback ensure # disconnect from server dbh.disconnect if dbh end
DELETE Operation
DELETE operation is required when you want to delete some records from your database. Following is the procedure to delete all the records from EMPLOYEE where AGE is more than 20. This operation will take following steps.
- Preparing SQL query based on required conditions. This will be done using the prepare method.
- Executing SQL query to delete required records from the database. This will be done using the execute method.
- Releasing Statement handle. This will be done using the finish method.
- If everything goes fine then commit this operation otherwise you can rollback the complete transaction.
#!/usr/bin/ruby -w require "dbi" begin # connect to the MySQL server dbh = DBI.connect("DBI:Mysql:TESTDB:localhost", "testuser", "test123") sth = dbh.prepare("DELETE FROM EMPLOYEE WHERE AGE > ?") sth.execute(20) sth.finish dbh.commit rescue DBI::DatabaseError => e puts "An error occurred" puts "Error code: #{e.err}" puts "Error message: #{e.errstr}" dbh.rollback ensure # disconnect from server dbh.disconnect if dbh end
Performing Transactions
Transactions are a mechanism that ensures data consistency. Transactions should have the following four properties −
- Atomicity − Either a transaction completes or nothing happens at all.
- Consistency − A transaction must start in a consistent state and leave the system is a consistent state.
- Isolation − Intermediate results of a transaction are not visible outside the current transaction.
- Durability − Once a transaction was committed, the effects are persistent, even after a system failure.
The DBI provides two methods to either commit or rollback a transaction. There is one more method called transaction which can be used to implement transactions. There are two simple approaches to implement transactions −
Approach I
The first approach uses DBI’s commit and rollback methods to explicitly commit or cancel the transaction −
dbh['AutoCommit'] = false # Set auto commit to false. begin dbh.do("UPDATE EMPLOYEE SET AGE = AGE+1 WHERE FIRST_NAME = 'John'") dbh.do("UPDATE EMPLOYEE SET AGE = AGE+1 WHERE FIRST_NAME = 'Zara'") dbh.commit rescue puts "transaction failed" dbh.rollback end dbh['AutoCommit'] = true
Approach II
The second approach uses the transaction method. This is simpler, because it takes a code block containing the statements that make up the transaction. The transaction method executes the block, then invokes commit or rollback automatically, depending on whether the block succeeds or fails −
dbh['AutoCommit'] = false # Set auto commit to false. dbh.transaction do |dbh| dbh.do("UPDATE EMPLOYEE SET AGE = AGE+1 WHERE FIRST_NAME = 'John'") dbh.do("UPDATE EMPLOYEE SET AGE = AGE+1 WHERE FIRST_NAME = 'Zara'") end dbh['AutoCommit'] = true
COMMIT Operation
Commit is the operation, which gives a green signal to database to finalize the changes, and after this operation, no change can be reverted back.
Here is a simple example to call the commit method.
dbh.commit
ROLLBACK Operation
If you are not satisfied with one or more of the changes and you want to revert back those changes completely, then use the rollback method.
Here is a simple example to call the rollback method.
dbh.rollback
Disconnecting Database
To disconnect Database connection, use disconnect API.
dbh.disconnect
If the connection to a database is closed by the user with the disconnect method, any outstanding transactions are rolled back by the DBI. However, instead of depending on any of DBI’s implementation details, your application would be better off calling the commit or rollback explicitly.
Handling Errors
There are many sources of errors. A few examples are a syntax error in an executed SQL statement, a connection failure, or calling the fetch method for an already canceled or finished statement handle.
If a DBI method fails, DBI raises an exception. DBI methods may raise any of several types of exception but the two most important exception classes are DBI::InterfaceError and DBI::DatabaseError.
Exception objects of these classes have three attributes named err, errstr, and state, which represent the error number, a descriptive error string, and a standard error code. The attributes are explained below −
- err − Returns an integer representation of the occurred error or nil if this is not supported by the DBD.The Oracle DBD for example returns the numerical part of an ORA-XXXX error message.
- errstr − Returns a string representation of the occurred error.
- state − Returns the SQLSTATE code of the occurred error.The SQLSTATE is a five-character-long string. Most DBDs do not support this and return nil instead.
You have seen following code above in most of the examples −
rescue DBI::DatabaseError => e puts "An error occurred" puts "Error code: #{e.err}" puts "Error message: #{e.errstr}" dbh.rollback ensure # disconnect from server dbh.disconnect if dbh end
To get debugging information about what your script is doing as it executes, you can enable tracing. To do this, you must first load the dbi/trace module and then call the trace method that controls the trace mode and output destination −
require "dbi/trace" .............. trace(mode, destination)
The mode value may be 0 (off), 1, 2, or 3, and the destination should be an IO object. The default values are 2 and STDERR, respectively.
Code Blocks with Methods
There are some methods that create handles. These methods can be invoked with a code block. The advantage of using code block along with methods is that they provide the handle to the code block as its parameter and automatically cleans up the handle when the block terminates. There are few examples to understand the concept.
- DBI.connect − This method generates a database handle and it is recommended to call disconnect at the end of the block to disconnect the database.
- dbh.prepare − This method generates a statement handle and it is recommended to finish at the end of the block. Within the block, you must invoke execute method to execute the statement.
- dbh.execute − This method is similar except we don’t need to invoke execute within the block. The statement handle is automatically executed.
Example 1
DBI.connect can take a code block, passes the database handle to it, and automatically disconnects the handle at the end of the block as follows.
dbh = DBI.connect("DBI:Mysql:TESTDB:localhost", "testuser", "test123") do |dbh|
Example 2
dbh.prepare can take a code block, passes the statement handle to it, and automatically calls finish at the end of the block as follows.
dbh.prepare("SHOW DATABASES") do |sth| sth.execute puts "Databases: " + sth.fetch_all.join(", ") end
Example 3
dbh.execute can take a code block, passes the statement handle to it, and automatically calls finish at the end of the block as follows −
dbh.execute("SHOW DATABASES") do |sth| puts "Databases: " + sth.fetch_all.join(", ") end
DBI transaction method also takes a code block which has been described in above.
Driver-specific Functions and Attributes
The DBI lets the database drivers provide additional database-specific functions, which can be called by the user through the func method of any Handle object.
Driver-specific attributes are supported and can be set or gotten using the []= or [] methods.
Example
#!/usr/bin/ruby require "dbi" begin # connect to the MySQL server dbh = DBI.connect("DBI:Mysql:TESTDB:localhost", "testuser", "test123") puts dbh.func(:client_info) puts dbh.func(:client_version) puts dbh.func(:host_info) puts dbh.func(:proto_info) puts dbh.func(:server_info) puts dbh.func(:thread_id) puts dbh.func(:stat) rescue DBI::DatabaseError => e puts "An error occurred" puts "Error code: #{e.err}" puts "Error message: #{e.errstr}" ensure dbh.disconnect if dbh end
This will produce the following result −
5.0.45 50045 Localhost via UNIX socket 10 5.0.45 150621 Uptime: 384981 Threads: 1 Questions: 1101078 Slow queries: 4 \ Opens: 324 Flush tables: 1 Open tables: 64 \ Queries per second avg: 2.860
Install Ruby on Windows:
Using RubyInstaller
To use RubyInstaller, you need to first download it from their downloads page. Notice that the list of options has “with Devkit” and “without Devkit” sections. The Devkit versions install the MSYS2 system, which will be required if you need to install RubyGems that require compilation. If you’re just going to play around with plain old Ruby, you might be able to get away with the non-Devkit version. But why limit yourself? If you want to work on a Ruby on Rails app, you’ll definitely need to compile gems, so you need the Devkit.
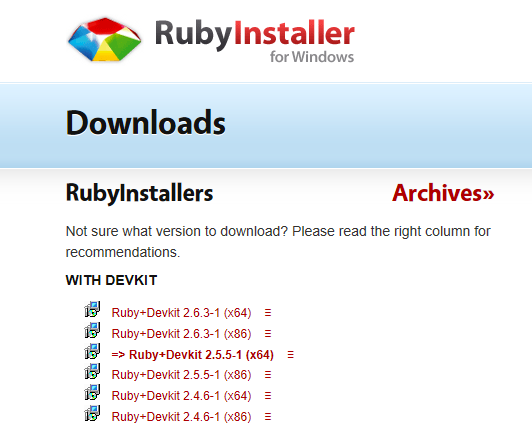
Also note that there are various Ruby versions available, and there are both x86 and x64 packages. Currently, there’s an arrow pointing at the 2.5.5-1 (x64) package. That’s because it’s still the preferred Ruby version for most people. Ruby 2.6.3 is still fairly new, so it won’t have as many gems available. Also, as it says on the page, “the 32 bit (x86) version is recommended only if custom 32-bit native DLLs or COM objects have to be used.” You should stick with x64.
Download that and run it to get started. You’ll first need to accept the license agreement.
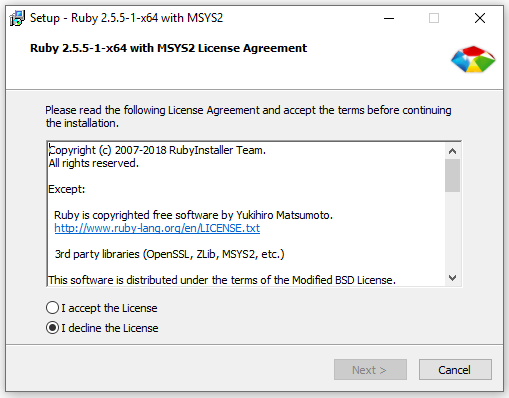
On the next screen, be sure to check the MSYS2 development toolchain option:

Then click “Next” and grab a cold one. It takes a few minutes to install everything.
Tooling up with the MSYS2 toolchain
Once you’ve done that, another important checkbox appears on the final screen of the installer: “Run ‘ridk install’ to set up MSYS2.” The GUI installer won’t do that part for you, so use this checkbox to kick that off next:
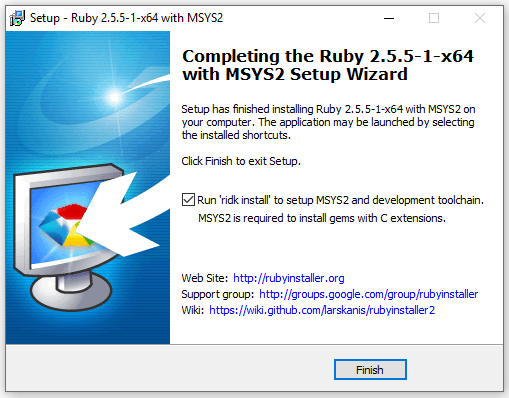
Installing the development toolchain is a command-line process, so a terminal window will open to complete this part:
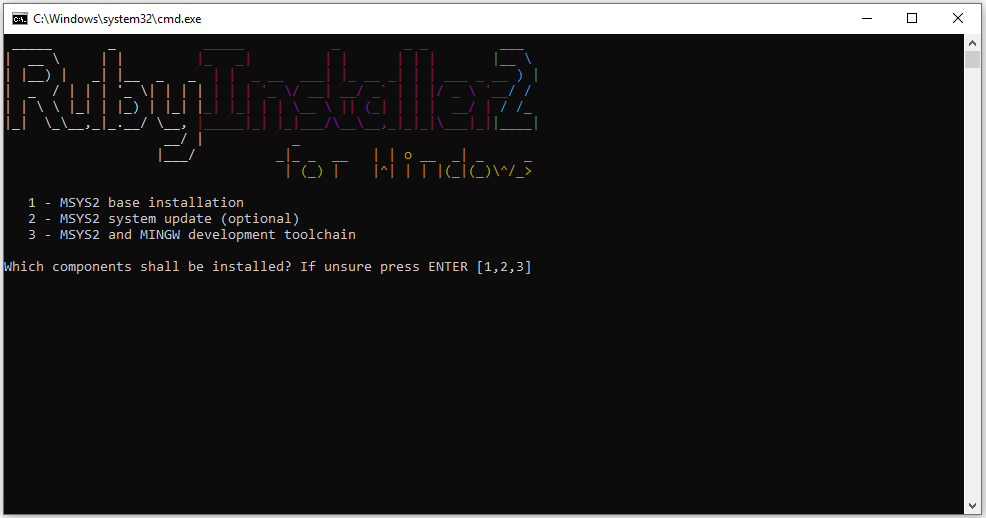
This “ridk install” command asks which components you want to install. It’s fine to just hit “Enter” to accept the default. This will install the base MSYS2 system, check for updates to it, and then install the development toolchain.
Once that’s rolling, you’ll see a lot of output streaming by. It takes at least as long as installing Ruby did. When you’ve done that, you should see the message “install MSYS2 and MinGW development toolchain succeeded.” Oddly enough, that leaves you at the same install prompt. Just hit “Enter” again to get out of that. The terminal window will close.
Ready to rock Ruby on Windows!
In order to check out your fresh Ruby install, you need to open a terminal window again. You can do so by opening the Windows menu and typing “cmd” in the search box. At the command prompt, type “ruby -v” and hit “Enter.” You should see something like this:
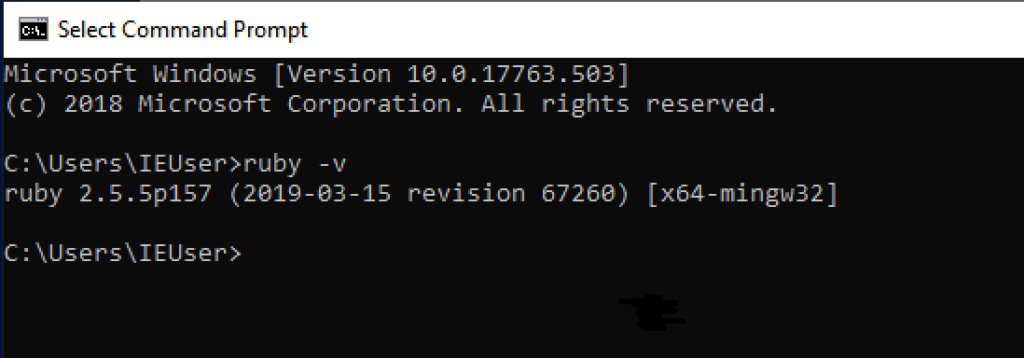
And running the command “gem list” will show you what RubyGems came with your fresh install:
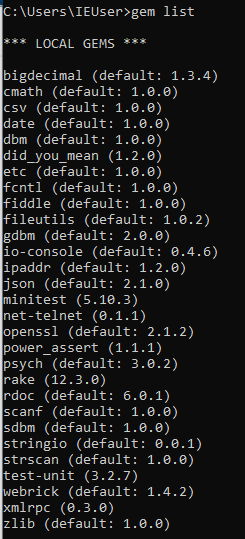
You can also run “irb,” the interactive Ruby shell:
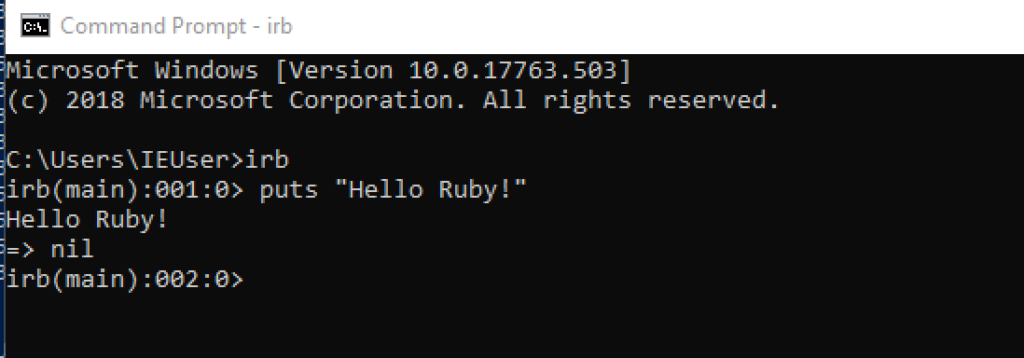
Try out some Ruby!
—Ruby is owned by Ruby (https://www.ruby-lang.org/en/) and they own all related trademarks and IP rights for this software.
Cognosys provides hardened images of Ruby on all public cloud ( AWS marketplace and Azure).
Ruby on Cloud runs on Amazon Web Services (AWS) and Azure and is built to provide intuitive and easy to use interface for publishing websites.
Secured Ruby on centos 7.3
Secured Ruby on Ubuntu 14.04 LTS
Ruby on cloud For Azure
Features
Features of Ruby
⦁ Object Oriented: Every values in Ruby is an object, even the most primitive things like strings, numbers and even true and false. So, Ruby is a pure Object-Oriented Language. Every object has a class and every class has one superclass.
⦁ Dynamic : Ruby is a very dynamic programming language. Ruby programs aren’t compiled like C or Java programs. All things in a program are built by the code when it is run. A program can also modify its own definitions while running.
⦁ Singleton Classes : Every object in Ruby has two classes: a regular class and a singleton class. An object’s singleton class is nameless class whose only instances is that object. Singleton classes are created automatically and make Ruby simple and elegant.
⦁ Metaprogramming : Everything in Ruby are objects. You can use them to learn about them or even modify them, while your program is running. This technique is called metaprogramming.
⦁ Flexibility : Methods can be added to existing classes without sub classing, operators can be overloaded, and even the behaviour of the standard library can be redefined at runtime.
⦁ Variables and scopes : Programmer do not need to declare variables or variable scope in ruby. The name of the variable automatically determines its scope.
Features of Ruby
Ruby language has many features. Some of them are explained below:
- Object-oriented
- Flexibility
- Expressive feature
- Mixins
- Visual appearance
- Dynamic typing and Duck typing
- Exception handling
- Garbage collector
- Portable
- Keywords
- Statement delimiters
- Variable constants
- Naming conventions
- Keyword arguments
- Method names
- Singleton methods
- Missing method
- Case Sensitive
Object Oriented
Ruby is purely object oriented programming language. Each and every value is an object. Every object has a class and every class has a super class. Every code has their properties and actions. Ruby is influenced with Smalltalk language. Rules applying to objects applies to the entire Ruby.
Flexibility
Ruby is a flexible language as you can easily remove, redefine or add existing parts to it. It allows its users to freely alter its parts as they wish.
Mixins
Ruby has a feature of single inheritance only. Ruby has classes as well as modules. A module has methods but no instances. Instead, a module can be mixed into a class, which adds the method of that module to the class. It is similar to inheritance but much more flexible.
Visual appearance
Ruby generally prefers English keyword and some punctuation is used to decorate Ruby. It doesn’t need variable declaration.
Dynamic typing and Duck typing
Ruby is a dynamic programming language. Ruby programs are not compiled. All class, module and method definition are built by the code when it run.Ruby variables are loosely typed language, which means any variable can hold any type of object. When a method is called on an object, Ruby only looks up at the name irrespective of the type of object. This is duck typing. It allows you to make classes that pretend to be other classes.
Variable constants
In Ruby, constants are not really constant. If an already initialized constant will be modified in a script, it will simply trigger a warning but will not halt your program.
Naming conventions
Ruby defines some naming conventions for its variable, method, constant and class.
- Constant: Starts with a capital letter.
- Global variable: Starts with a dollar sign ($).
- Instance variable: Starts with a (@) sign.
- Class variable: Starts with a (@@) sign.
- Method name: Allowed to start with a capital letter.
Keyword arguments
Like Python, Ruby methods can also be defined using keyword arguments.
Method names
Methods are allowed to end with question mark (?) or exclamation mark (!). By convention, methods that answer questions end with question mark and methods that indicates that method can change the state of the object end with exclamation mark.
Singleton methods
Ruby singleton methods are per-object methods. They are only available on the object you defined it on.
Missing method
If a method is lost, Ruby calls the method_missing method with name of the lost method.
Statement delimiters
Multiple statements in a single line must contain semi colon in between but not at the end of a line.
Keywords
In Ruby there are approximately 42 keywords which can’t be used for other purposes. They are called reserved words.
Case Sensitive
Ruby is a case-sensitive language. Lowercase letters and uppercase letters are different.
-Major Features of Ruby
- Ruby is an open-source and is freely available on the Web, but it is subject to a license.
- Ruby is a general-purpose, interpreted programming language.
- Ruby is a true object-oriented programming language.
- Ruby is a server-side scripting language similar to Python and PERL.
- Ruby can be used to write Common Gateway Interface (CGI) scripts.
- Ruby can be embedded into Hypertext Markup Language (HTML).
- Ruby has a clean and easy syntax that allows a new developer to learn Ruby very quickly and easily.
Azure
Installation Instructions For Ubuntu
Note : How to find PublicDNS in Azure
Step 1) SSH Connection: To connect to the deployed instance, Please follow Instructions to Connect to Ubuntu instance on Azure Cloud
1) Download Putty.
2) Connect to virtual machine using following SSH credentials:
- Hostname: PublicDNS / IP of machine
- Port : 22
Username: Your chosen username when you created the machine ( For example: Azureuser)
Password : Your Chosen Password when you created the machine ( How to reset the password if you do not remember)
Step 2) Application URL: Access the application via a browser at “http://PublicDNS/”.
Step 3) Other Information:
1. Default installation path: will be in your web root folder “/usr/bin/ruby”.
2. Default ports:
- Linux Machines: SSH Port – 22 or 2222
- Http: 80 or 8080
- Https: 443
Configure custom inbound and outbound rules using this link
3. To access Webmin interface for management please follow this link
Installation Instructions For Centos
Note : How to find PublicDNS in Azure
Step 1) SSH Connection: To connect to the deployed instance, Please follow Instructions to Connect to Centos instance on Azure Cloud
1) Download Putty.
2) Connect to virtual machine using following SSH credentials:
- Hostname: PublicDNS / IP of machine
- Port : 22
Username: Your chosen username when you created the machine ( For example: Azureuser)
Password : Your Chosen Password when you created the machine ( How to reset the password if you do not remember)
Step 3) Application URL: Access the application via a browser at “http://PublicDNS/”
Step 4) Other Information:
1. Default installation path: will be on your web root folder “/opt/Ruby”
2.Default ports:
- Linux Machines: SSH Port – 22
- Http: 80
- Https: 443
Configure custom inbound and outbound rules using this link
3. To access Webmin interface for management please follow this link
Azure Step by Step Screenshots
Product Name
Pricing Details